
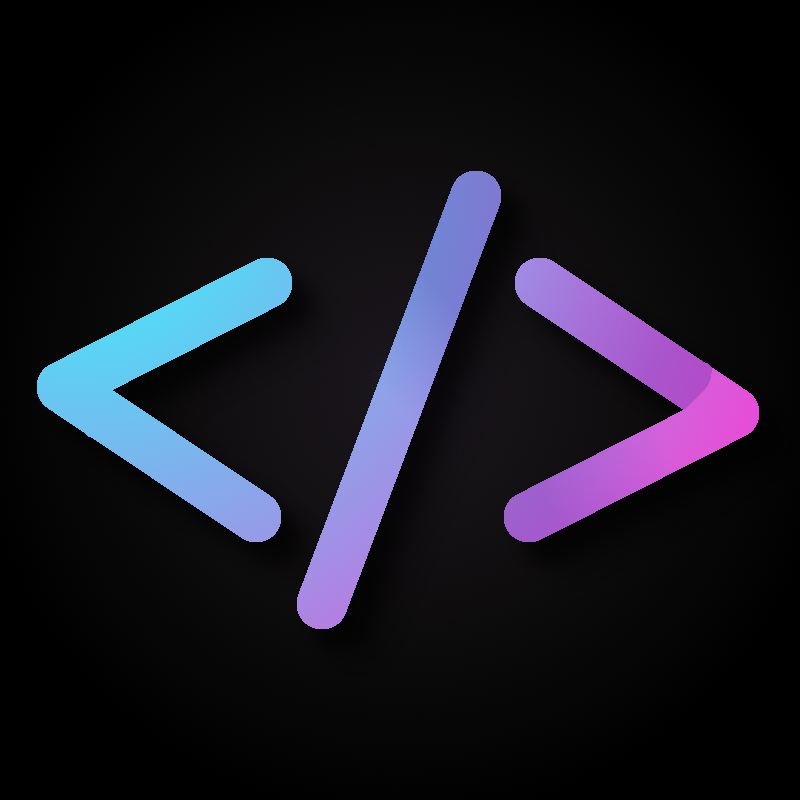
This shit is why I cannot recommend Truffle/Graal. Yes, it’s cool technology. Yes, it works well. Yes, I remember Chris Seaton. Yes, most of it is Free Software. However, Oracle is still the fucking lawnmower, and it’s not safe to build upon anything they can convince a judge they might own.
Alternatives include RPython (my preference) and also GNU Lightning.
I think they’re saying that e.g. you shouldn’t index a natural key unless you know that you’re going to search/collate by that key as a column. Telling the database that a certain column contains (a component of) the primary key is adding a restriction to that column.